1. Overview
This portfolio documents my contributions to the project I have undertaken in the course CS2103T - Software Engineering. It aims to demonstrate the theoretical and practical knowledge I have gained in the field of Software Engineering over the semester.
2. Project
TopDeck is a general purpose flashcard application created to solve the inherent problems associated with physical flashcards. It aims to facilitate quick and intuitive management of virtual flashcards as well as having a study mode which allows for extra-efficient studying. It comprises three management systems - 1) Deck, 2) Card, 3) Study, each of which plays an indispensable role in ensuring the application’s success. They were meticulously designed to be simplistic and intuitive to use.
3. Summary of Contributions
This section acts as a summary of my contributions to TopDeck.
Enhancement added: I added the deck list to allow for the management of decks and also the ability to import and export decks.
-
What it does: The deck list allows users to better navigate and manage decks. It allows users to add, edit and find decks with ease. The ability to import and export whole decks removes the resource-intensive way of having to manually create decks and cards.
-
Justification: In order for a flash card application to work seamlessly, it needs the ability to manage and store cards easily. This deck management system allows the user to store related cards into decks. Importing also allows users to download and import pre-made decks for quick and easy use. Exporting allows for portability and also the sharing of decks between different users.
Code Contributed: My GitHub commits, My RepoSense Dashboard
Other contributions:
-
Project management:
-
Ensured loose coupling between deck and card management.
-
Ensured Storage component of TopDeck preserved functionality throughout.
-
-
Enhancements to existing features:
-
Quality Assurance:
-
Documentation:
-
Community:
-
I reviewed pull requests for decks and cards.
-
4. Contributions to User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
4.1. Decks view
In this view you can create, edit and find decks. This is the default view when TopDeck is first launched.
These are commands only available in deck view.
4.1.1. Creating a deck: add
Format: add n/DECK_NAME
Outcome: Creates a new deck called DECK_NAME
.
Example: add n/History
Here is what you type in.
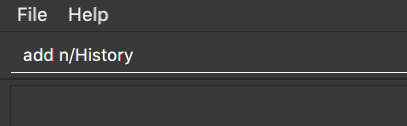
The deck should appear at the bottom of the list.
Before:
After:
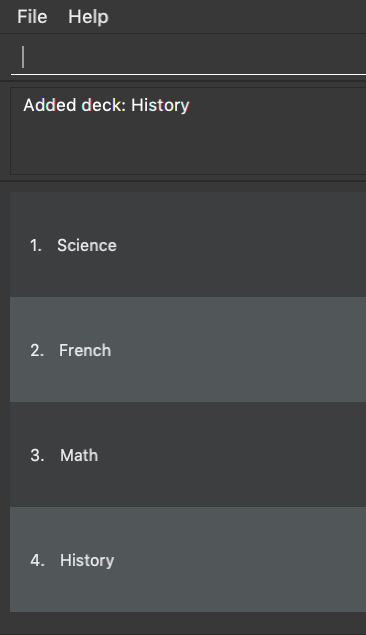
4.1.2. Deleting a deck: delete
Format: delete INDEX
Outcome: Deletes the deck at INDEX
.
Example: delete 2
The index refers to the index number shown in the displayed deck list. The index must be a positive integer 1, 2, 3… |
Before:
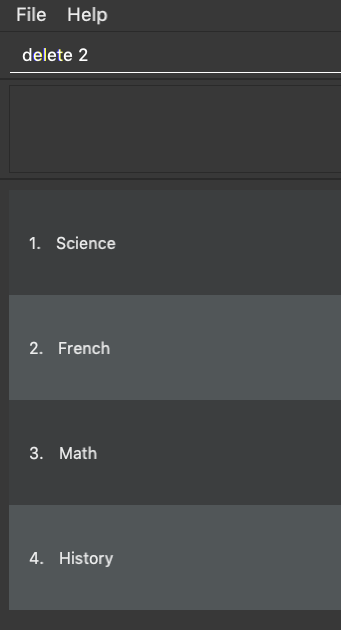
After:
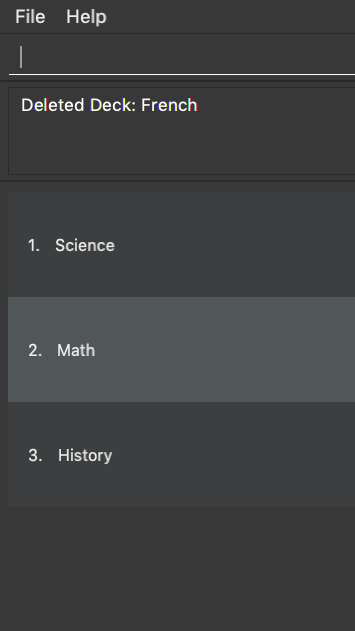
4.1.3. Editing the name of a deck: edit
Format: edit INDEX n/NEW_DECK_NAME
Outcome: Changes the name of the deck at INDEX
to NEW_DECK_NAME
.
Example: edit 2 n/Addition
Before:
.png)
After:
.png)
4.1.4. Finding a deck by name: find
Format: find KEYWORD
Outcome: Lists all decks containing KEYWORD
in its name.
Example: find sci
Before:
.png)
After:
.png)
4.1.6. Navigating into a deck: open
Format: open INDEX
Outcome: Opens the deck at INDEX
Example: open 1
.png)
4.1.7. Studying a deck: study
Format: study INDEX
Outcome: Enters study view with the deck at INDEX
.
Example: study 1
.png)
4.1.8. Import a deck: import
To import a deck from the json
file at the specified FILEPATH.
Format: import FILEPATH
Example: Say you want to import a deck called "Economics" and you have the Economics.json file in the same folder as TopDeck.jar.
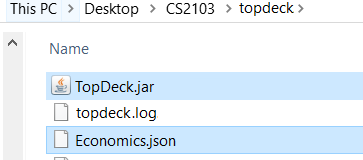
-
Simply enter
import Economics
and TopDeck will import the deck "Economics".
Before:
.png)
After:
.png)
4.1.9. Export a deck: export
To create a json
file of the deck at INDEX.
Format: export INDEX
Example:
-
1. First, display all the decks in TopDeck using
list
.
.png)
-
2. Say you want to export "History" (the 3rd deck), simply enter the command:
export 3
. You should see the following message:

"Economics.json" will be created in the same directory as the TopDeck.jar file.
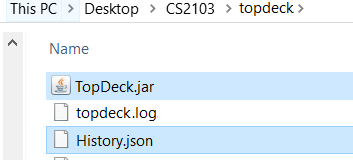
4.1.10. Selecting a deck: select
Currently select does not do much. However, we will be adding more functionality for select in v2.0. Refer to [Upcoming Features] for more details.
5. Contributions to Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
5.1. Deck operations
5.1.1. Current implementation
Deck operations are supported in TopDeck class: A Deck consists of a list of cards
. Decks are deemed as equal if they
have the same name. This is to prevent users from creating 2 or more decks with the same name.
Within the Model, Deck
is encapsulated by the following data structure:
-
Model
-
VersionedTopDeck
-
TopDeck
-
UniqueDeckList
-
Deck
The Create, Read, Update and Delete(CRUD) operation will trickle down the encapsulations
and be executed in UniqueDeckList
.
5.1.2. Current implementation
Deck Management is facilitated by Deck
which implements the following operations:
-
add(Deck deck)
-
edit(Deck target, Deck editedDeck)
-
delete(Deck deck)
-
find(Name name)
The CRUD operations are exposed in the Model interface as Model#addDeck(Deck deck)
and
Model#deleteDeck(Deck toDelete)
. For each deck operation,
there are 2 main updates that need to be done. The first update will be on the model
and the second will be on the ViewState
.
Given below is an example usage scenario and how the addDeck(Deck) mechanism behaves at each step:
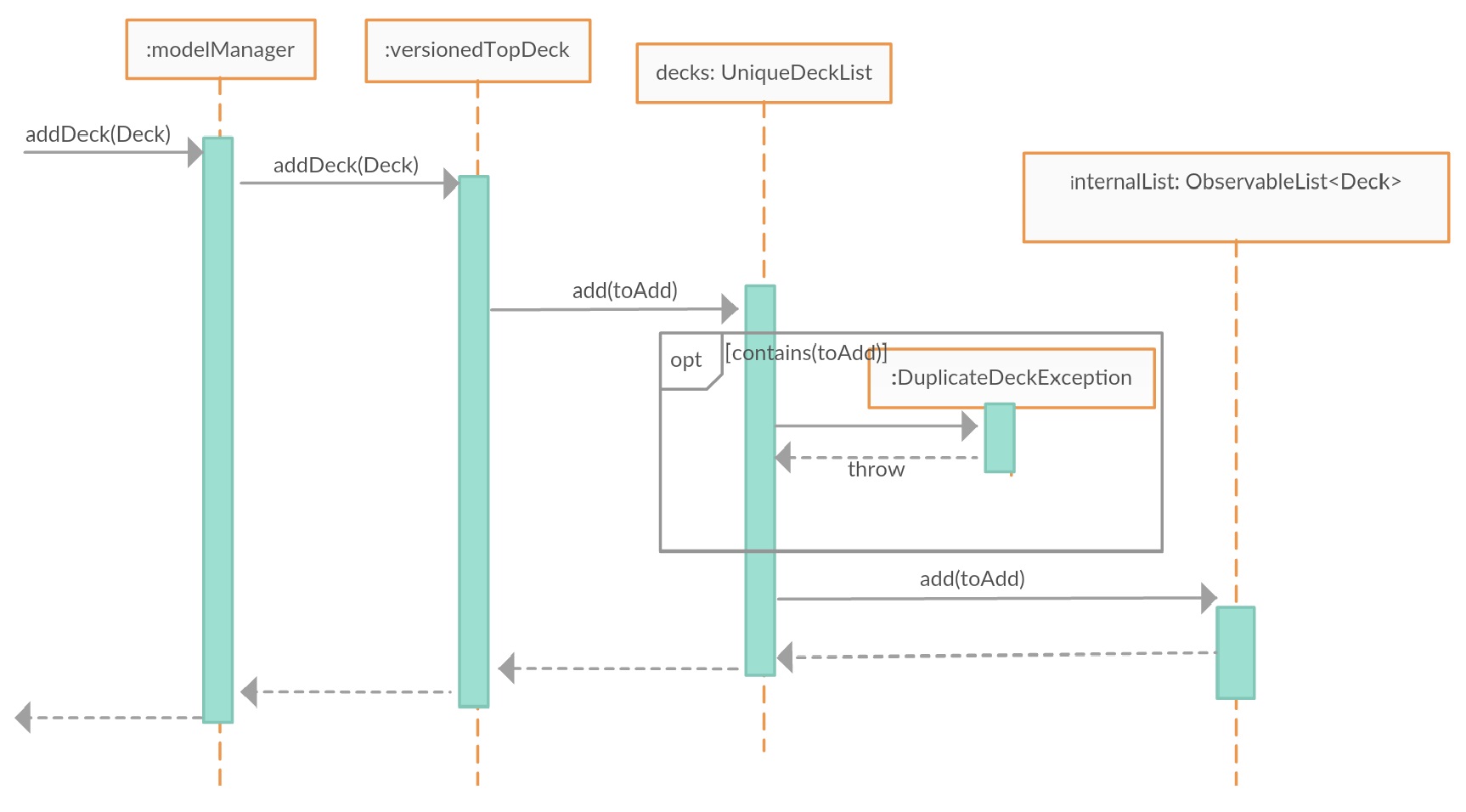
-
The user starts up the application and is in the
DecksView
. The user then executes theadd
commandadd n/NAME
to create a new deck. Theadd
command is parsed and callsModel#addDeck(Deck deck)
. -
Model#addDeck(Deck deck)
first checks if the current state is a DecksView. Following, it will create a new deck to be added into `VersionedTopDeck.addDeck(Deck deck). -
Once that is done, the filteredItems list is being updated to reflect the change.
-
To continue to add
cards
, the user will then execute the commandselect INDEX
. For example, user executes theselect 1
command to select the first deck. This should change theViewState
in theModelManager
fromDeckView
toCardView
. For more information oncards
, refer to cards’s feature.
5.1.3. Design considerations
-
Alternative 1 (current choice): Implement the logic of deck operations in TopDeck class.
-
Pros: Easy to implement and debug as all logic related with executing commands are implemented in TopDeck.
-
Cons: Card class is not informed, or notified when its UniqueDeckList is modified. This might result in unexpected behaviors if a deck command is executed and the person in charge of Card class assumes that the UniqueDeckList is unmodified.
-
-
Alternative 2: Implement the logic of card-level operations in Deck class.
-
Pros: The responsibility of each class is clear, only a Deck can modify its list of cards.
-
Cons: The logic for executing deck-level and card-level commands are implemented at different places. We must ensure that the implementation of each command is correct.
-
-
Why Alternative 1: Without changing the current Undo/Redo feature makes it difficult to implement as we have decided to go with a stateful implementation. However being stateful allows for more features like our study mode in the future. The following design considerations for card management will better illustrate why we decided to go with this approach.